Note
Go to the end to download the full example code.
Maxwell 3D: magnet DC analysis#
This example shows how you can use PyAEDT to create a Maxwell DC analysis, compute mass center, and move coordinate systems.
Perform required imports#
Perform required imports.
from pyaedt import Maxwell3d
from pyaedt import generate_unique_project_name
import os
import tempfile
Set AEDT version#
Set AEDT version.
aedt_version = "2024.1"
Create temporary directory#
Create temporary directory.
temp_dir = tempfile.TemporaryDirectory(suffix=".ansys")
Set non-graphical mode#
Set non-graphical mode.
You can set non_graphical
either to True
or False
.
non_graphical = False
Launch AEDT#
Launch AEDT in graphical mode.
m3d = Maxwell3d(project=generate_unique_project_name(),
version=aedt_version,
new_desktop=True,
non_graphical=non_graphical)
C:\actions-runner\_work\_tool\Python\3.10.9\x64\lib\subprocess.py:1072: ResourceWarning: subprocess 5716 is still running
_warn("subprocess %s is still running" % self.pid,
C:\actions-runner\_work\pyaedt\pyaedt\.venv\lib\site-packages\pyaedt\generic\settings.py:428: ResourceWarning: unclosed file <_io.TextIOWrapper name='D:\\Temp\\pyaedt_ansys.log' mode='a' encoding='cp1252'>
self._logger = val
Set up Maxwell solution#
Set up the Maxwell solution to DC.
m3d.solution_type = m3d.SOLUTIONS.Maxwell3d.ElectroDCConduction
Create magnet#
Create a magnet.
magnet = m3d.modeler.create_box(origin=[7, 4, 22], sizes=[10, 5, 30], name="Magnet", material="copper")
Create setup and assign voltage#
Create the setup and assign a voltage.
m3d.assign_voltage(magnet.faces, 0)
m3d.create_setup()
SetupName MySetupAuto with 0 Sweeps
Plot model#
Plot the model.
m3d.plot(show=False, output_file=os.path.join(temp_dir.name, "Image.jpg"), plot_air_objects=True)
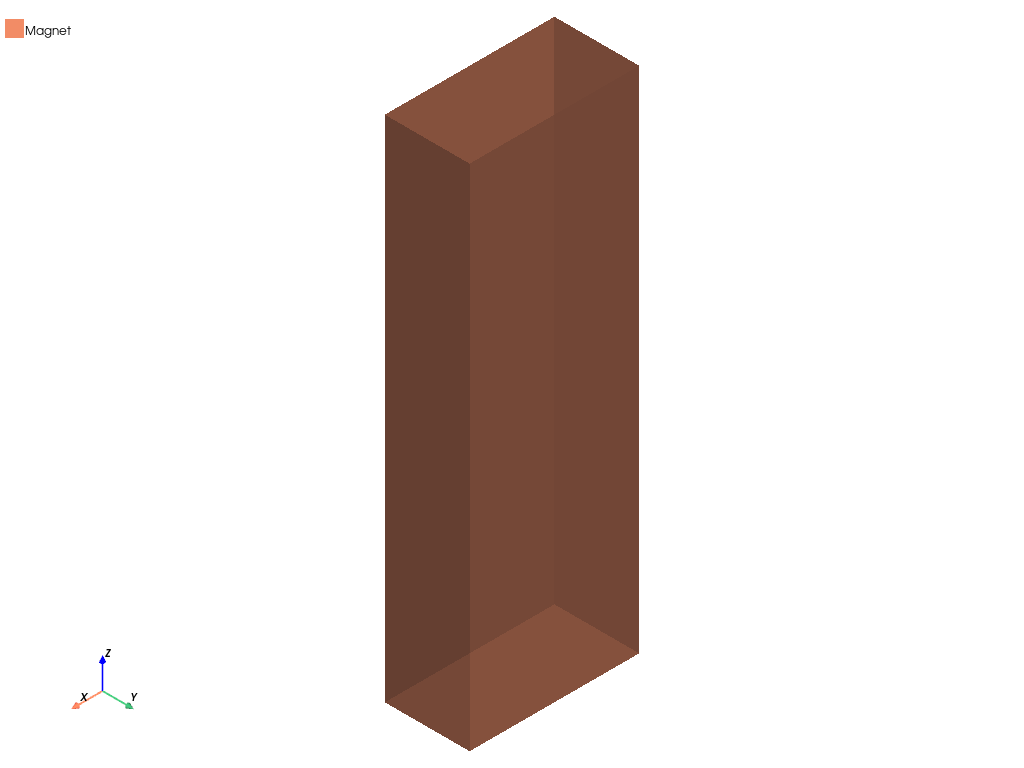
<pyaedt.generic.plot.ModelPlotter object at 0x00000187CAC724D0>
Solve setup#
Solve the setup.
m3d.analyze()
True
Compute mass center#
Compute mass center using the fields calculator.
m3d.post.ofieldsreporter.EnterScalarFunc("X")
m3d.post.ofieldsreporter.EnterVol(magnet.name)
m3d.post.ofieldsreporter.CalcOp("Mean")
m3d.post.ofieldsreporter.AddNamedExpression("CM_X", "Fields")
m3d.post.ofieldsreporter.EnterScalarFunc("Y")
m3d.post.ofieldsreporter.EnterVol(magnet.name)
m3d.post.ofieldsreporter.CalcOp("Mean")
m3d.post.ofieldsreporter.AddNamedExpression("CM_Y", "Fields")
m3d.post.ofieldsreporter.EnterScalarFunc("Z")
m3d.post.ofieldsreporter.EnterVol(magnet.name)
m3d.post.ofieldsreporter.CalcOp("Mean")
m3d.post.ofieldsreporter.AddNamedExpression("CM_Z", "Fields")
m3d.post.ofieldsreporter.CalcStack("clear")
Get mass center#
Get mass center using the fields calculator.
Create variables#
Create variables with mass center values.
Create coordinate system#
Create a parametric coordinate system.
cs1 = m3d.modeler.create_coordinate_system(
[magnet.name + "x", magnet.name + "y", magnet.name + "z"], reference_cs="Global", name=magnet.name + "CS"
)
Save and close#
Save the project and close AEDT.
m3d.save_project()
m3d.release_desktop(close_projects=True, close_desktop=True)
temp_dir.cleanup()
Total running time of the script: (0 minutes 56.014 seconds)