Note
Go to the end to download the full example code.
Maxwell 3D: choke setup#
This example shows how you can use PyAEDT to create a choke setup in Maxwell 3D.
Perform required imports#
Perform required imports.
import json
import os
import pyaedt
import tempfile
Set AEDT version#
Set AEDT version.
aedt_version = "2024.1"
Create temporary directory#
Create temporary directory.
temp_dir = tempfile.TemporaryDirectory(suffix=".ansys")
Set non-graphical mode#
Set non-graphical mode.
You can define non_graphical
either to True
or False
.
non_graphical = False
Launch Maxwell3D#
Launch Maxwell 3D 2023 R2 in graphical mode.
m3d = pyaedt.Maxwell3d(project=pyaedt.generate_unique_project_name(),
solution_type="EddyCurrent",
version=aedt_version,
non_graphical=non_graphical,
new_desktop=True
)
C:\actions-runner\_work\_tool\Python\3.10.9\x64\lib\subprocess.py:1072: ResourceWarning: subprocess 5072 is still running
_warn("subprocess %s is still running" % self.pid,
C:\actions-runner\_work\pyaedt\pyaedt\.venv\lib\site-packages\pyaedt\generic\settings.py:428: ResourceWarning: unclosed file <_io.TextIOWrapper name='D:\\Temp\\pyaedt_ansys.log' mode='a' encoding='cp1252'>
self._logger = val
Rules and information of use#
The dictionary values containing the different parameters of the core and
the windings that compose the choke. You must not change the main structure of
the dictionary. The dictionary has many primary keys, including
"Number of Windings"
, "Layer"
, and "Layer Type"
, that have
dictionaries as values. The keys of these dictionaries are secondary keys
of the dictionary values, such as "1"
, "2"
, "3"
, "4"
, and
"Simple"
.
You must not modify the primary or secondary keys. You can modify only their values.
You must not change the data types for these keys. For the dictionaries from
"Number of Windings"
through "Wire Section"
, values must be Boolean. Only
one value per dictionary can be "True"
. If all values are True
, only the first one
remains set to True
. If all values are False
, the first value is chosen as the
correct one by default. For the dictionaries from "Core"
through "Inner Winding"
,
values must be strings, floats, or integers.
Descriptions follow for primary keys:
"Number of Windings"
: Number of windings around the core"Layer"
: Number of layers of all windings"Layer Type"
: Whether layers of a winding are linked to each other"Similar Layer"
: Whether layers of a winding have the same number of turns and same spacing between turns"Mode"
: When there are only two windows, whether they are in common or differential mode"Wire Section"
: Type of wire section and number of segments"Core"
: Design of the core"Outer Winding"
: Design of the first layer or outer layer of a winding and the common parameters for all layers"Mid Winding"
: Turns and turns spacing (“Coil Pit”) for the second or mid layer if it is necessary"Inner Winding"
: Turns and turns spacing (“Coil Pit”) for the third or inner layer if it is necessary"Occupation(%)"
: An informative parameter that is useless to modify
The following parameter values work. You can modify them if you want.
values = {
"Number of Windings": {"1": False, "2": False, "3": True, "4": False},
"Layer": {"Simple": False, "Double": False, "Triple": True},
"Layer Type": {"Separate": False, "Linked": True},
"Similar Layer": {"Similar": False, "Different": True},
"Mode": {"Differential": True, "Common": False},
"Wire Section": {"None": False, "Hexagon": False, "Octagon": True, "Circle": False},
"Core": {
"Name": "Core",
"Material": "ferrite",
"Inner Radius": 100,
"Outer Radius": 143,
"Height": 25,
"Chamfer": 0.8,
},
"Outer Winding": {
"Name": "Winding",
"Material": "copper",
"Inner Radius": 100,
"Outer Radius": 143,
"Height": 25,
"Wire Diameter": 5,
"Turns": 2,
"Coil Pit(deg)": 4,
"Occupation(%)": 0,
},
"Mid Winding": {"Turns": 7, "Coil Pit(deg)": 4, "Occupation(%)": 0},
"Inner Winding": {"Turns": 10, "Coil Pit(deg)": 4, "Occupation(%)": 0},
}
Convert dictionary to JSON file#
Covert a dictionary to a JSON file. PyAEDT methods ask for the path of the JSON file as an argument. You can convert a dictionary to a JSON file.
json_path = os.path.join(temp_dir.name, "choke_example.json")
with open(json_path, "w") as outfile:
json.dump(values, outfile)
Verify parameters of JSON file#
Verify parameters of the JSON file. The check_choke_values
method takes
the JSON file path as an argument and does the following:
Checks if the JSON file is correctly written (as explained in the rules)
Checks inequations on windings parameters to avoid having unintended intersections
dictionary_values = m3d.modeler.check_choke_values(json_path, create_another_file=False)
print(dictionary_values)
[True, {'Number of Windings': {'1': False, '2': False, '3': True, '4': False}, 'Layer': {'Simple': False, 'Double': False, 'Triple': True}, 'Layer Type': {'Separate': False, 'Linked': True}, 'Similar Layer': {'Similar': False, 'Different': True}, 'Mode': {'Differential': True, 'Common': False}, 'Wire Section': {'None': False, 'Hexagon': False, 'Octagon': True, 'Circle': False}, 'Core': {'Name': 'Core', 'Material': 'ferrite', 'Inner Radius': 100, 'Outer Radius': 143, 'Height': 25, 'Chamfer': 0.8}, 'Outer Winding': {'Name': 'Winding', 'Material': 'copper', 'Inner Radius': 86.25, 'Outer Radius': 156.75, 'Height': 52.5, 'Wire Diameter': 5, 'Turns': 2, 'Coil Pit(deg)': 4, 'Occupation(%)': 13.333333333333334}, 'Mid Winding': {'Turns': 7, 'Coil Pit(deg)': 4.0, 'Occupation(%)': 46.666666666666664}, 'Inner Winding': {'Turns': 10, 'Coil Pit(deg)': 4.0, 'Occupation(%)': 66.66666666666667}}]
Create choke#
Create the choke. The create_choke
method takes the JSON file path as an
argument.
list_object = m3d.modeler.create_choke(json_path)
print(list_object)
core = list_object[1]
first_winding_list = list_object[2]
second_winding_list = list_object[3]
third_winding_list = list_object[4]
[<pyaedt.modeler.cad.object3d.Object3d object at 0x0000018804C26D40>, <pyaedt.modeler.cad.object3d.Object3d object at 0x0000018804C25ED0>, [<pyaedt.modeler.cad.polylines.Polyline object at 0x0000018804C274C0>, [[-74.497822093321, 62.511095042016, -52.5], [-74.497822093321, 62.511095042016, 12.839087296526], [-76.344688372662, 64.060799855845, 15.25], [-115.963757581084, 84.252601589961, 15.25], [-117.914226930149, 85.669700521628, 12.839087296526], [-117.914226930149, 85.669700521628, -12.839087296526], [-115.963757581084, 84.252601589961, -15.25], [-84.517247279393, 52.812237532635, -15.25], [-82.472677351212, 51.534648446679, -12.839087296526], [-82.472677351212, 51.534648446679, 12.839087296526], [-84.517247279393, 52.812237532635, 15.25], [-126.560902091063, 67.293625321403, 15.25], [-128.689611659189, 68.425480276044, 12.839087296526], [-128.689611659189, 68.425480276044, -12.839087296526], [-126.560902091063, 67.293625321403, -15.25], [-91.044774104774, 40.535745078881, -15.25], [-88.842295755743, 39.555138539122, -12.839087296526], [-88.842295755743, 39.555138539122, 12.839087296526], [-91.044774104774, 40.535745078881, 15.25], [-134.694682602733, 49.024855181328, 15.25], [-136.960199479546, 49.849435889716, 12.839087296526], [-136.960199479546, 49.849435889716, -12.839087296526], [-134.694682602733, 49.024855181328, -15.25], [-95.800217964102, 27.470270455894, -15.25], [-93.482699930001, 26.805732853203, -12.839087296526], [-93.482699930001, 26.805732853203, 12.839087296526], [-95.800217964102, 27.470270455894, 15.25], [-140.20678433047, 29.801872000095, 15.25], [-142.565012806952, 30.303128936688, 12.839087296526], [-142.565012806952, 30.303128936688, -12.839087296526], [-140.20678433047, 29.801872000095, -15.25], [-98.691019551891, 13.870118265453, -15.25], [-96.303569685118, 13.534584068366, -12.839087296526], [-96.303569685118, 13.534584068366, 12.839087296526], [-98.691019551891, 13.870118265453, 15.25], [-142.989920484069, 9.998829279507, 15.25], [-145.394960325369, 10.167006048206, 12.839087296526], [-145.394960325369, 10.167006048206, -12.839087296526], [-142.989920484069, 9.998829279507, -15.25], [-99.660912703474, 0.0, -15.25], [-97.25, 0.0, -12.839087296526], [-97.25, 0.0, 12.839087296526], [-99.660912703474, 0.0, 15.25], [-142.989920484069, -9.998829279507, 15.25], [-145.394960325369, -10.167006048206, 12.839087296526], [-145.394960325369, -10.167006048206, -12.839087296526], [-142.989920484069, -9.998829279507, -15.25], [-98.691019551891, -13.870118265453, -15.25], [-96.303569685118, -13.534584068366, -12.839087296526], [-96.303569685118, -13.534584068366, 12.839087296526], [-98.691019551891, -13.870118265453, 15.25], [-140.20678433047, -29.801872000095, 15.25], [-142.565012806952, -30.303128936688, 12.839087296526], [-142.565012806952, -30.303128936688, -12.839087296526], [-140.20678433047, -29.801872000095, -15.25], [-95.800217964102, -27.470270455894, -15.25], [-93.482699930001, -26.805732853203, -12.839087296526], [-93.482699930001, -26.805732853203, 12.839087296526], [-95.800217964102, -27.470270455894, 15.25], [-134.694682602733, -49.024855181328, 15.25], [-136.960199479546, -49.849435889716, 12.839087296526], [-136.960199479546, -49.849435889716, -12.839087296526], [-134.694682602733, -49.024855181328, -15.25], [-91.044774104774, -40.535745078881, -15.25], [-88.842295755743, -39.555138539122, -12.839087296526], [-88.842295755743, -39.555138539122, 12.839087296526], [-91.044774104774, -40.535745078881, 15.25], [-126.560902091063, -67.293625321403, 15.25], [-128.689611659189, -68.425480276044, 12.839087296526], [-128.689611659189, -68.425480276044, -12.839087296526], [-126.560902091063, -67.293625321403, -15.25], [-84.517247279393, -52.812237532635, -15.25], [-82.472677351212, -51.534648446679, -12.839087296526], [-82.472677351212, -51.534648446679, 12.839087296526], [-84.517247279393, -52.812237532635, 15.25], [-115.963757581084, -84.252601589961, 15.25], [-117.914226930149, -85.669700521628, 12.839087296526], [-117.914226930149, -85.669700521628, -12.839087296526], [-115.963757581084, -84.252601589961, -15.25], [-76.344688372662, -64.060799855845, -15.25], [-74.497822093321, -62.511095042016, -12.839087296526], [-74.497822093321, -62.511095042016, 15.117261889578], [-76.344688372662, -64.060799855845, 20.75], [-117.806839543016, -85.591679017905, 20.75], [-122.363820399211, -88.902519409237, 15.117261889578], [-122.363820399211, -88.902519409237, -15.117261889578], [-117.806839543016, -85.591679017905, -20.75], [-82.585245653043, -51.60498892849, -20.75], [-77.808412822352, -48.620092493397, -15.117261889578], [-77.808412822352, -48.620092493397, 15.117261889578], [-82.585245653043, -51.60498892849, 20.75], [-128.572410864111, -68.363163507903, 20.75], [-133.545823419913, -71.007573871366, 15.117261889578], [-133.545823419913, -71.007573871366, -15.117261889578], [-128.572410864111, -68.363163507903, -20.75], [-88.963558053575, -39.609127992563, -20.75], [-83.817795738709, -37.318087002205, -15.117261889578], [-83.817795738709, -37.318087002205, 15.117261889578], [-88.963558053575, -39.609127992563, 20.75], [-136.835466456686, -49.804036782165, 20.75], [-142.128508893869, -51.730546678007, 15.117261889578], [-142.128508893869, -51.730546678007, -15.117261889578], [-136.835466456686, -49.804036782165, -20.75], [-93.610295991141, -26.842320434976, -20.75], [-88.195760602341, -25.28972739621, -15.117261889578], [-88.195760602341, -25.28972739621, 15.117261889578], [-93.610295991141, -26.842320434976, 20.75], [-142.435175342717, -30.275531131715, 20.75], [-147.944824610988, -31.446643236186, 15.117261889578], [-147.944824610988, -31.446643236186, -15.117261889578], [-142.435175342717, -30.275531131715, -20.75], [-96.435015997374, -13.553057642809, -20.75], [-90.857095307039, -12.769132013086, -15.117261889578], [-90.857095307039, -12.769132013086, 15.117261889578], [-96.435015997374, -13.553057642809, 20.75], [-145.262545558313, -10.157746705692, 20.75], [-150.881562601798, -10.550666653799, 15.117261889578], [-150.881562601798, -10.550666653799, -15.117261889578], [-145.262545558313, -10.157746705692, -20.75], [-97.382738110422, 0.0, -20.75], [-91.75, 0.0, -15.117261889578], [-91.75, 0.0, 15.117261889578], [-97.382738110422, 0.0, 20.75], [-145.262545558313, 10.157746705692, 20.75], [-150.881562601798, 10.550666653799, 15.117261889578], [-150.881562601798, 10.550666653799, -15.117261889578], [-145.262545558313, 10.157746705692, -20.75], [-96.435015997374, 13.553057642809, -20.75], [-90.857095307039, 12.769132013086, -15.117261889578], [-90.857095307039, 12.769132013086, 15.117261889578], [-96.435015997374, 13.553057642809, 20.75], [-142.435175342717, 30.275531131715, 20.75], [-147.944824610988, 31.446643236186, 15.117261889578], [-147.944824610988, 31.446643236186, -15.117261889578], [-147.944824610988, 31.446643236186, -17.39543648263], [-142.435175342717, 30.275531131715, -26.25], [-94.179012442856, 13.235997020166, -26.25], [-85.41062092896, 12.003679957806, -17.39543648263], [-85.41062092896, 12.003679957806, 17.39543648263], [-94.179012442856, 13.235997020166, 26.25], [-147.535170632557, 10.316664131877, 26.25], [-156.368164878227, 10.934327259392, 17.39543648263], [-156.368164878227, 10.934327259392, -17.39543648263], [-147.535170632557, 10.316664131877, -26.25], [-95.10456351737, 0.0, -26.25], [-86.25, 0.0, -17.39543648263], [-86.25, 0.0, 17.39543648263], [-95.10456351737, 0.0, 26.25], [-147.535170632557, -10.316664131877, 26.25], [-156.368164878227, -10.934327259392, 17.39543648263], [-156.368164878227, -10.934327259392, -17.39543648263], [-156.368164878227, -10.934327259392, -52.5]]], [<pyaedt.modeler.cad.polylines.Polyline object at 0x0000018804C279D0>, [[-16.887285278109, -95.772553980438, -52.5], [-16.887285278109, -95.772553980438, 12.839087296526], [-17.305935875581, -98.146839502654, 15.25], [-14.983014521293, -142.553860778499, 15.25], [-15.23502352126, -144.951566249926, 12.839087296526], [-15.23502352126, -144.951566249926, -12.839087296526], [-14.983014521293, -142.553860778499, -15.25], [-3.478115694263, -99.600201968203, -15.25], [-3.393976054318, -97.190757927607, -12.839087296526], [-3.393976054318, -97.190757927607, 12.839087296526], [-3.478115694263, -99.600201968203, 15.25], [5.002462004445, -143.251768997437, 15.25], [5.086601644389, -145.661213038034, 12.839087296526], [5.086601644389, -145.661213038034, -12.839087296526], [5.002462004445, -143.251768997437, -15.25], [10.417402052746, -99.11495979599, -15.25], [10.165393052779, -96.717254324565, -12.839087296526], [10.165393052779, -96.717254324565, 12.839087296526], [10.417402052746, -99.11495979599, 15.25], [24.890571297483, -141.161444479313, 15.25], [25.309221894955, -143.535730001529, 12.839087296526], [25.309221894955, -143.535730001529, -12.839087296526], [24.890571297483, -141.161444479313, -15.25], [24.110156918418, -96.700557672946, -15.25], [23.526904347068, -94.36125938034, -12.839087296526], [23.526904347068, -94.36125938034, 12.839087296526], [24.110156918418, -96.700557672946, 15.25], [44.294213932821, -136.32357301316, 15.25], [45.039226930149, -138.616487250018, 12.839087296526], [45.039226930149, -138.616487250018, -12.839087296526], [44.294213932821, -136.32357301316, -15.25], [37.333635004569, -92.403989190051, -15.25], [36.430491209698, -90.16862985662, -12.839087296526], [36.430491209698, -90.16862985662, 12.839087296526], [37.333635004569, -92.403989190051, 15.25], [62.835720077878, -128.832318264074, 15.25], [63.892594644508, -130.999232248103, 12.839087296526], [63.892594644508, -130.999232248103, -12.839087296526], [62.835720077878, -128.832318264074, -15.25], [49.830456351737, -86.308882165552, -15.25], [48.625, -84.220970518037, -12.839087296526], [48.625, -84.220970518037, 12.839087296526], [49.830456351737, -86.308882165552, 15.25], [80.154200406191, -118.833488984567, 15.25], [81.502365680861, -120.832226199897, 12.839087296526], [81.502365680861, -120.832226199897, -12.839087296526], [80.154200406191, -118.833488984567, -15.25], [61.357384547322, -78.533870924598, -15.25], [59.87307847542, -76.634045788254, -12.839087296526], [59.87307847542, -76.634045788254, 12.839087296526], [61.357384547322, -78.533870924598, 15.25], [95.912570397649, -106.521701013065, 15.25], [97.525785876803, -108.31335831333, 12.839087296526], [97.525785876803, -108.31335831333, -12.839087296526], [95.912570397649, -106.521701013065, -15.25], [71.690061045684, -69.230287217052, -15.25], [69.955795582933, -67.555526527137, -12.839087296526], [69.955795582933, -67.555526527137, 12.839087296526], [71.690061045684, -69.230287217052, 15.25], [109.80411130525, -92.136589297985, 15.25], [111.650977584591, -93.686294111813, 12.839087296526], [111.650977584591, -93.686294111813, -12.839087296526], [109.80411130525, -92.136589297985, -15.25], [80.627372052028, -58.579214717109, -15.25], [78.676902702964, -57.162115785443, -12.839087296526], [78.676902702964, -57.162115785443, 12.839087296526], [80.627372052028, -58.579214717109, 15.25], [121.558440086618, -75.958143676034, 15.25], [123.6030100148, -77.23573276199, 12.839087296526], [123.6030100148, -77.23573276199, -12.839087296526], [121.558440086618, -75.958143676034, -15.25], [87.995362973656, -46.787964435568, -15.25], [85.86665340553, -45.656109480928, -12.839087296526], [85.86665340553, -45.656109480928, 12.839087296526], [87.995362973656, -46.787964435568, 15.25], [130.946772102377, -58.301259188539, 15.25], [133.149250451409, -59.281865728298, 12.839087296526], [133.149250451409, -59.281865728298, -12.839087296526], [130.946772102377, -58.301259188539, -15.25], [93.650624248243, -34.086039646809, -15.25], [91.38510737143, -33.261458938422, -12.839087296526], [91.38510737143, -33.261458938422, 15.117261889578], [93.650624248243, -34.086039646809, 20.75], [133.027988153577, -59.227876274857, 20.75], [138.173750468444, -61.518917265215, 15.117261889578], [138.173750468444, -61.518917265215, -15.117261889578], [133.027988153577, -59.227876274857, -20.75], [85.983854200609, -45.718426249069, -20.75], [81.010441644807, -43.074015885605, -15.117261889578], [81.010441644807, -43.074015885605, 15.117261889578], [85.983854200609, -45.718426249069, 20.75], [123.490441712969, -77.165392280179, 20.75], [128.26727454366, -80.150288715273, 15.117261889578], [128.26727454366, -80.150288715273, -15.117261889578], [123.490441712969, -77.165392280179, -20.75], [78.784290090096, -57.240137289166, -20.75], [74.227309233902, -53.929296897835, -15.117261889578], [74.227309233902, -53.929296897835, 15.117261889578], [78.784290090096, -57.240137289166, 20.75], [111.549294292712, -93.600971699101, 20.75], [115.864222021745, -97.22162596509, 15.117261889578], [115.864222021745, -97.22162596509, -15.117261889578], [111.549294292712, -93.600971699101, -20.75], [70.051279388782, -67.647734166621, -20.75], [65.999426681072, -63.734905489613, -15.117261889578], [65.999426681072, -63.734905489613, 15.117261889578], [70.051279388782, -67.647734166621, 20.75], [97.43696674449, -108.214714673426, 20.75], [101.206004211777, -112.400654853456, 15.117261889578], [101.206004211777, -112.400654853456, -15.117261889578], [97.43696674449, -108.214714673426, -20.75], [59.954800216314, -76.73864484668, -20.75], [56.486940361129, -72.299986643417, -15.117261889578], [56.486940361129, -72.299986643417, 15.117261889578], [59.954800216314, -76.73864484668, 20.75], [81.428139471493, -120.722181319047, 20.75], [84.57792664995, -125.39193284895, 15.117261889578], [84.57792664995, -125.39193284895, -15.117261889578], [81.428139471493, -120.722181319047, -20.75], [48.691369055211, -84.335925093712, -20.75], [45.875, -79.457830797222, -15.117261889578], [45.875, -79.457830797222, 15.117261889578], [48.691369055211, -84.335925093712, 20.75], [63.83440608682, -130.879928024739, 20.75], [66.303635951848, -135.942599502749, 15.117261889578], [66.303635951848, -135.942599502749, -15.117261889578], [63.83440608682, -130.879928024739, -20.75], [36.48021578106, -90.291702489489, -20.75], [34.37015494591, -85.069118656503, -15.117261889578], [34.37015494591, -85.069118656503, 15.117261889578], [36.48021578106, -90.291702489489, 20.75], [44.998208598227, -138.490245805141, 20.75], [46.738820399211, -143.847298089642, 15.117261889578], [46.738820399211, -143.847298089642, -15.117261889578], [46.738820399211, -143.847298089642, -17.39543648263], [44.998208598227, -138.490245805141, -26.25], [35.626796557549, -88.179415788927, -26.25], [32.309818682122, -79.969607456385, -17.39543648263], [32.309818682122, -79.969607456385, 17.39543648263], [35.626796557549, -88.179415788927, 26.25], [64.833092095761, -132.927537785405, 26.25], [68.714677259187, -140.885966757394, 17.39543648263], [68.714677259187, -140.885966757394, -17.39543648263], [64.833092095761, -132.927537785405, -26.25], [47.552281758685, -82.362968021873, -26.25], [43.125, -74.694691076408, -17.39543648263], [43.125, -74.694691076408, 17.39543648263], [47.552281758685, -82.362968021873, 26.25], [82.702078536796, -122.610873653528, 26.25], [87.65348761904, -129.951639498002, 17.39543648263], [87.65348761904, -129.951639498002, -17.39543648263], [87.65348761904, -129.951639498002, -52.5]]], [<pyaedt.modeler.cad.polylines.Polyline object at 0x0000018804C24760>, [[91.38510737143, 33.261458938422, -52.5], [91.38510737143, 33.261458938422, 12.839087296526], [93.650624248243, 34.086039646809, 15.25], [130.946772102377, 58.301259188538, 15.25], [133.149250451409, 59.281865728298, 12.839087296526], [133.149250451409, 59.281865728298, -12.839087296526], [130.946772102377, 58.301259188538, -15.25], [87.995362973656, 46.787964435568, -15.25], [85.86665340553, 45.656109480928, -12.839087296526], [85.86665340553, 45.656109480928, 12.839087296526], [87.995362973656, 46.787964435568, 15.25], [121.558440086618, 75.958143676034, 15.25], [123.6030100148, 77.23573276199, 12.839087296526], [123.6030100148, 77.23573276199, -12.839087296526], [121.558440086618, 75.958143676034, -15.25], [80.627372052028, 58.579214717109, -15.25], [78.676902702964, 57.162115785443, -12.839087296526], [78.676902702964, 57.162115785443, 12.839087296526], [80.627372052028, 58.579214717109, 15.25], [109.80411130525, 92.136589297985, 15.25], [111.650977584591, 93.686294111813, 12.839087296526], [111.650977584591, 93.686294111813, -12.839087296526], [109.80411130525, 92.136589297985, -15.25], [71.690061045684, 69.230287217052, -15.25], [69.955795582933, 67.555526527137, -12.839087296526], [69.955795582933, 67.555526527137, 12.839087296526], [71.690061045684, 69.230287217052, 15.25], [95.912570397649, 106.521701013065, 15.25], [97.525785876803, 108.31335831333, 12.839087296526], [97.525785876803, 108.31335831333, -12.839087296526], [95.912570397649, 106.521701013065, -15.25], [61.357384547322, 78.533870924598, -15.25], [59.87307847542, 76.634045788254, -12.839087296526], [59.87307847542, 76.634045788254, 12.839087296526], [61.357384547322, 78.533870924598, 15.25], [80.154200406191, 118.833488984567, 15.25], [81.502365680861, 120.832226199897, 12.839087296526], [81.502365680861, 120.832226199897, -12.839087296526], [80.154200406191, 118.833488984567, -15.25], [49.830456351737, 86.308882165552, -15.25], [48.625, 84.220970518037, -12.839087296526], [48.625, 84.220970518037, 12.839087296526], [49.830456351737, 86.308882165552, 15.25], [62.835720077878, 128.832318264074, 15.25], [63.892594644508, 130.999232248103, 12.839087296526], [63.892594644508, 130.999232248103, -12.839087296526], [62.835720077878, 128.832318264074, -15.25], [37.333635004569, 92.403989190051, -15.25], [36.430491209698, 90.16862985662, -12.839087296526], [36.430491209698, 90.16862985662, 12.839087296526], [37.333635004569, 92.403989190051, 15.25], [44.294213932821, 136.32357301316, 15.25], [45.039226930149, 138.616487250018, 12.839087296526], [45.039226930149, 138.616487250018, -12.839087296526], [44.294213932821, 136.32357301316, -15.25], [24.110156918418, 96.700557672946, -15.25], [23.526904347068, 94.36125938034, -12.839087296526], [23.526904347068, 94.36125938034, 12.839087296526], [24.110156918418, 96.700557672946, 15.25], [24.890571297483, 141.161444479313, 15.25], [25.309221894955, 143.535730001529, 12.839087296526], [25.309221894955, 143.535730001529, -12.839087296526], [24.890571297483, 141.161444479313, -15.25], [10.417402052746, 99.11495979599, -15.25], [10.165393052779, 96.717254324565, -12.839087296526], [10.165393052779, 96.717254324565, 12.839087296526], [10.417402052746, 99.11495979599, 15.25], [5.002462004445, 143.251768997437, 15.25], [5.086601644389, 145.661213038034, 12.839087296526], [5.086601644389, 145.661213038034, -12.839087296526], [5.002462004445, 143.251768997437, -15.25], [-3.478115694263, 99.600201968203, -15.25], [-3.393976054318, 97.190757927607, -12.839087296526], [-3.393976054318, 97.190757927607, 12.839087296526], [-3.478115694263, 99.600201968203, 15.25], [-14.983014521293, 142.5538607785, 15.25], [-15.23502352126, 144.951566249926, 12.839087296526], [-15.23502352126, 144.951566249926, -12.839087296526], [-14.983014521293, 142.5538607785, -15.25], [-17.305935875581, 98.146839502654, -15.25], [-16.887285278109, 95.772553980438, -12.839087296526], [-16.887285278109, 95.772553980438, 15.117261889578], [-17.305935875581, 98.146839502654, 20.75], [-15.221148610561, 144.819555292762, 20.75], [-15.809930069233, 150.421436674452, 15.117261889578], [-15.809930069233, 150.421436674452, -15.117261889578], [-15.221148610561, 144.819555292762, -20.75], [-3.398608547565, 97.323415177559, -20.75], [-3.202028822455, 91.694108379002, -15.117261889578], [-3.202028822455, 91.694108379002, 15.117261889578], [-3.398608547565, 97.323415177559, 20.75], [5.081969151142, 145.528555788082, 20.75], [5.278548876253, 151.157862586639, 15.117261889578], [5.278548876253, 151.157862586639, -15.117261889578], [5.081969151142, 145.528555788082, -20.75], [10.179267963479, 96.849265281729, -20.75], [9.590486504807, 91.24738390004, -15.117261889578], [9.590486504807, 91.24738390004, 15.117261889578], [10.179267963479, 96.849265281729, 20.75], [25.286172163974, 143.405008481266, 20.75], [26.264286872124, 148.952172643097, 15.117261889578], [26.264286872124, 148.952172643097, -15.117261889578], [25.286172163974, 143.405008481266, -20.75], [23.559016602359, 94.490054601597, -20.75], [22.196333921269, 89.024632885823, -15.117261889578], [22.196333921269, 89.024632885823, 15.117261889578], [23.559016602359, 94.490054601597, 20.75], [44.998208598227, 138.490245805141, 20.75], [46.738820399211, 143.847298089642, 15.117261889578], [46.738820399211, 143.847298089642, -15.117261889578], [44.998208598227, 138.490245805141, -20.75], [36.48021578106, 90.291702489489, -20.75], [34.37015494591, 85.069118656503, -15.117261889578], [34.37015494591, 85.069118656503, 15.117261889578], [36.48021578106, 90.291702489489, 20.75], [63.83440608682, 130.879928024739, 20.75], [66.303635951848, 135.942599502749, 15.117261889578], [66.303635951848, 135.942599502749, -15.117261889578], [63.83440608682, 130.879928024739, -20.75], [48.691369055211, 84.335925093712, -20.75], [45.875, 79.457830797222, -15.117261889578], [45.875, 79.457830797222, 15.117261889578], [48.691369055211, 84.335925093712, 20.75], [81.428139471494, 120.722181319047, 20.75], [84.57792664995, 125.39193284895, 15.117261889578], [84.57792664995, 125.39193284895, -15.117261889578], [81.428139471494, 120.722181319047, -20.75], [59.954800216314, 76.73864484668, -20.75], [56.486940361129, 72.299986643417, -15.117261889578], [56.486940361129, 72.299986643417, 15.117261889578], [59.954800216314, 76.73864484668, 20.75], [97.43696674449, 108.214714673426, 20.75], [101.206004211777, 112.400654853456, 15.117261889578], [101.206004211777, 112.400654853456, -15.117261889578], [101.206004211777, 112.400654853456, -17.39543648263], [97.43696674449, 108.214714673426, -26.25], [58.552215885307, 74.943418768761, -26.25], [53.100802246838, 67.965927498579, -17.39543648263], [53.100802246838, 67.965927498579, 17.39543648263], [58.552215885307, 74.943418768761, 26.25], [82.702078536796, 122.610873653528, 26.25], [87.65348761904, 129.951639498002, 17.39543648263], [87.65348761904, 129.951639498002, -17.39543648263], [82.702078536796, 122.610873653528, -26.25], [47.552281758685, 82.362968021873, -26.25], [43.125, 74.694691076408, -17.39543648263], [43.125, 74.694691076408, 17.39543648263], [47.552281758685, 82.362968021873, 26.25], [64.833092095761, 132.927537785405, 26.25], [68.714677259187, 140.885966757394, 17.39543648263], [68.714677259187, 140.885966757394, -17.39543648263], [68.714677259187, 140.885966757394, -52.5]]]]
Assign excitations#
Assign excitations.
first_winding_faces = m3d.modeler.get_object_faces(first_winding_list[0].name)
second_winding_faces = m3d.modeler.get_object_faces(second_winding_list[0].name)
third_winding_faces = m3d.modeler.get_object_faces(third_winding_list[0].name)
m3d.assign_current([first_winding_faces[-1]], amplitude=1000, phase="0deg", swap_direction=False, name="phase_1_in")
m3d.assign_current([first_winding_faces[-2]], amplitude=1000, phase="0deg", swap_direction=True, name="phase_1_out")
m3d.assign_current([second_winding_faces[-1]], amplitude=1000, phase="120deg", swap_direction=False, name="phase_2_in")
m3d.assign_current([second_winding_faces[-2]], amplitude=1000, phase="120deg", swap_direction=True, name="phase_2_out")
m3d.assign_current([third_winding_faces[-1]], amplitude=1000, phase="240deg", swap_direction=False, name="phase_3_in")
m3d.assign_current([third_winding_faces[-2]], amplitude=1000, phase="240deg", swap_direction=True, name="phase_3_out")
<pyaedt.modules.Boundary.BoundaryObject object at 0x000001880273A5C0>
Assign matrix#
Assign the matrix.
m3d.assign_matrix(["phase_1_in", "phase_2_in", "phase_3_in"], matrix_name="current_matrix")
<pyaedt.modules.Boundary.MaxwellParameters object at 0x0000018804C162C0>
Create mesh operation#
Create the mesh operation.
mesh = m3d.mesh
mesh.assign_skin_depth(assignment=[first_winding_list[0], second_winding_list[0], third_winding_list[0]],
skin_depth=0.20, triangulation_max_length="10mm", name="skin_depth")
mesh.assign_surface_mesh_manual([first_winding_list[0], second_winding_list[0], third_winding_list[0]],
surface_deviation=None, normal_dev="30deg", name="surface_approx")
<pyaedt.modules.Mesh.MeshOperation object at 0x000001880273B8B0>
Create boundaries#
Create the boundaries. A region with openings is needed to run the analysis.
region = m3d.modeler.create_air_region(x_pos=100, y_pos=100, z_pos=100, x_neg=100, y_neg=100, z_neg=0)
Create setup#
Create a setup with a sweep to run the simulation. Depending on your machine’s computing power, the simulation can take some time to run.
setup = m3d.create_setup("MySetup")
print(setup.props)
setup.props["Frequency"] = "100kHz"
setup.props["PercentRefinement"] = 15
setup.props["MaximumPasses"] = 10
setup.props["HasSweepSetup"] = True
setup.add_eddy_current_sweep(range_type="LinearCount", start=100, end=1000, count=12, units="kHz", clear=True)
SetupProps([('Enabled', True), ('MeshLink', SetupProps([('ImportMesh', False)])), ('MaximumPasses', 6), ('MinimumPasses', 1), ('MinimumConvergedPasses', 1), ('PercentRefinement', 30), ('SolveFieldOnly', False), ('PercentError', 1), ('SolveMatrixAtLast', True), ('UseIterativeSolver', False), ('RelativeResidual', 1e-05), ('NonLinearResidual', 0.0001), ('SmoothBHCurve', False), ('Frequency', '60Hz'), ('HasSweepSetup', False), ('SweepRanges', SetupProps([('Subrange', SetupProps([('SweepSetupType', 'LinearStep'), ('StartValue', '1e-08GHz'), ('StopValue', '1e-06GHz'), ('StepSize', '1e-08GHz')]))])), ('UseHighOrderShapeFunc', False), ('UseMuLink', False)])
True
Save project#
Save the project.
m3d.save_project()
m3d.modeler.fit_all()
m3d.plot(show=False, output_file=os.path.join(temp_dir.name, "Image.jpg"), plot_air_objects=True)
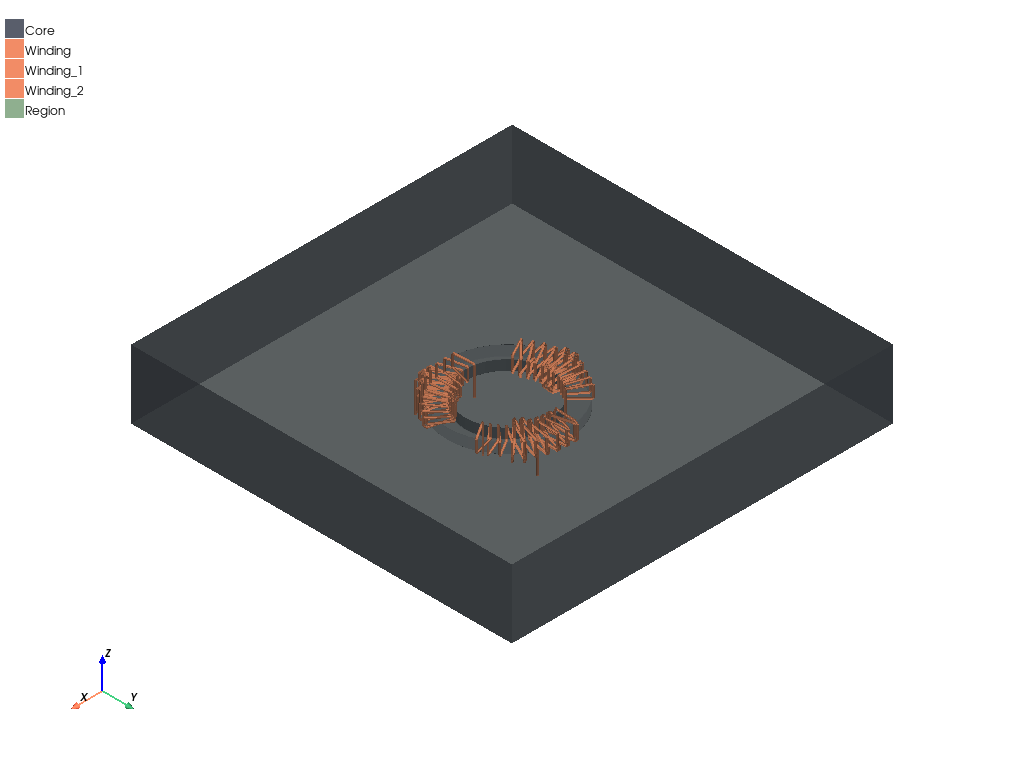
<pyaedt.generic.plot.ModelPlotter object at 0x0000018804C24FD0>
Close AEDT#
After the simulation completes, you can close AEDT or release it using the
pyaedt.Desktop.release_desktop()
method.
All methods provide for saving the project before closing.
m3d.release_desktop()
temp_dir.cleanup()
Total running time of the script: (0 minutes 55.964 seconds)