Visualization#
This section outlines the available modules for creating and editing data within and outside AEDT.
PyAEDT offers four primary levels of visualization:
Reports
Post-processing
Graphics
Advanced Visualization
Reports#
AEDT provides extensive flexibility for generating reports. PyAEDT includes dedicated classes to manipulate all report properties, offering full control over report customization.
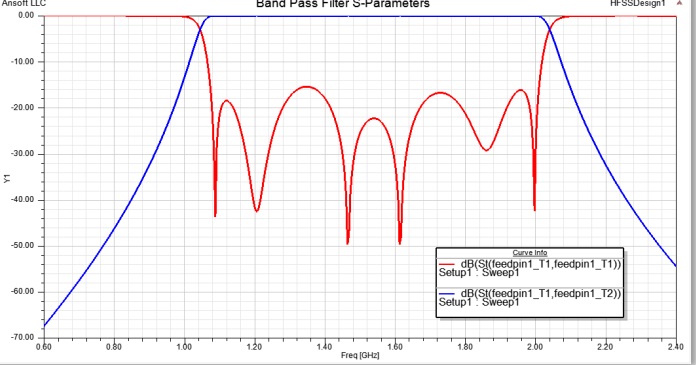
Post-processing#
AEDT has different post-processing tools. PyAEDT provides classes to interact with and modify any of these tools, enhancing data analysis and visualization capabilities.
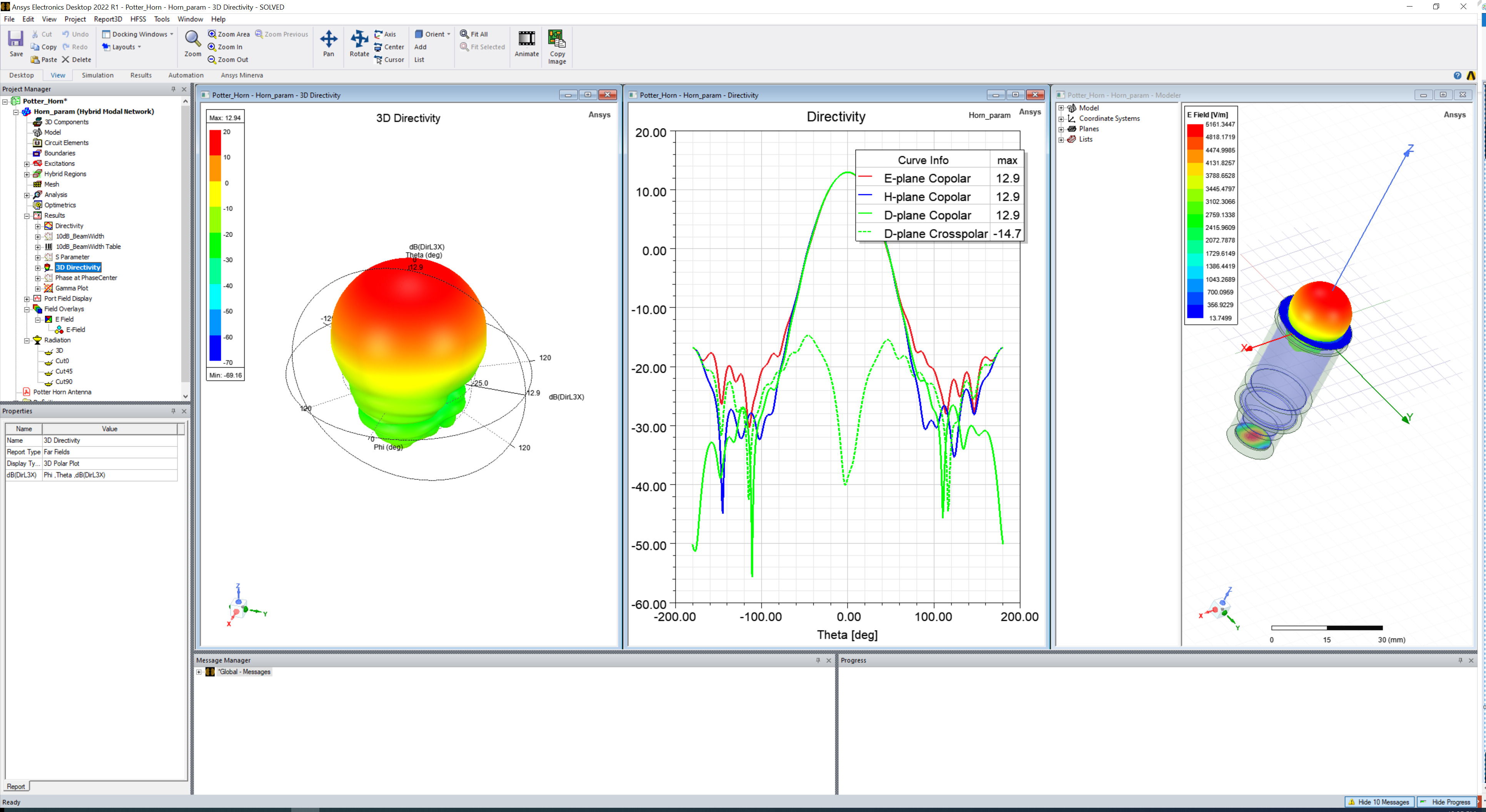
Graphics#
Specialized plotting options.
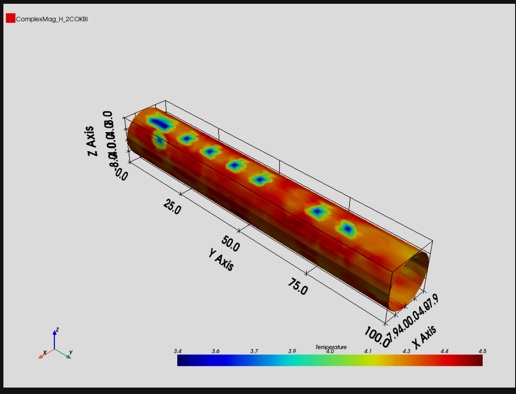
Advanced Visualization#
High-level visualization tools.
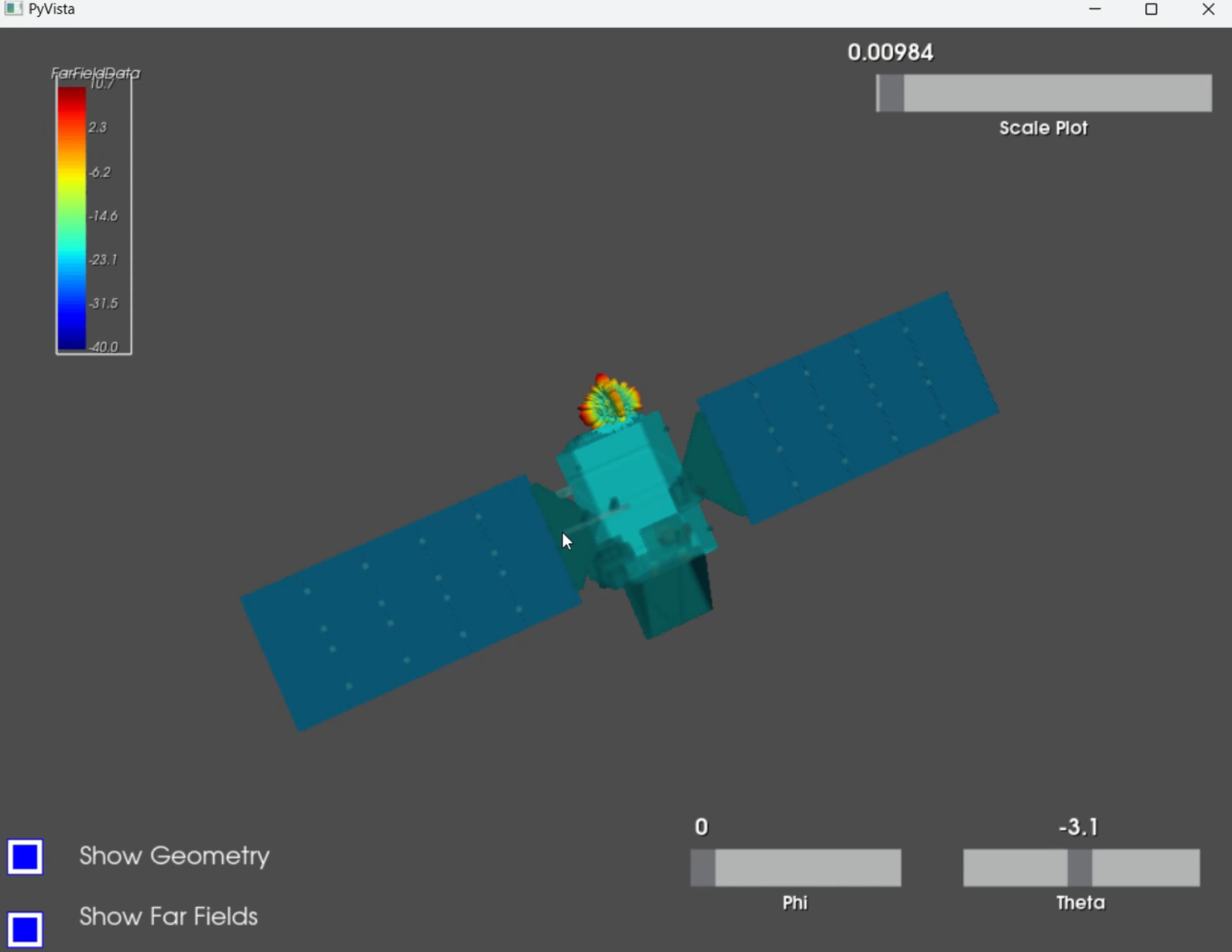